
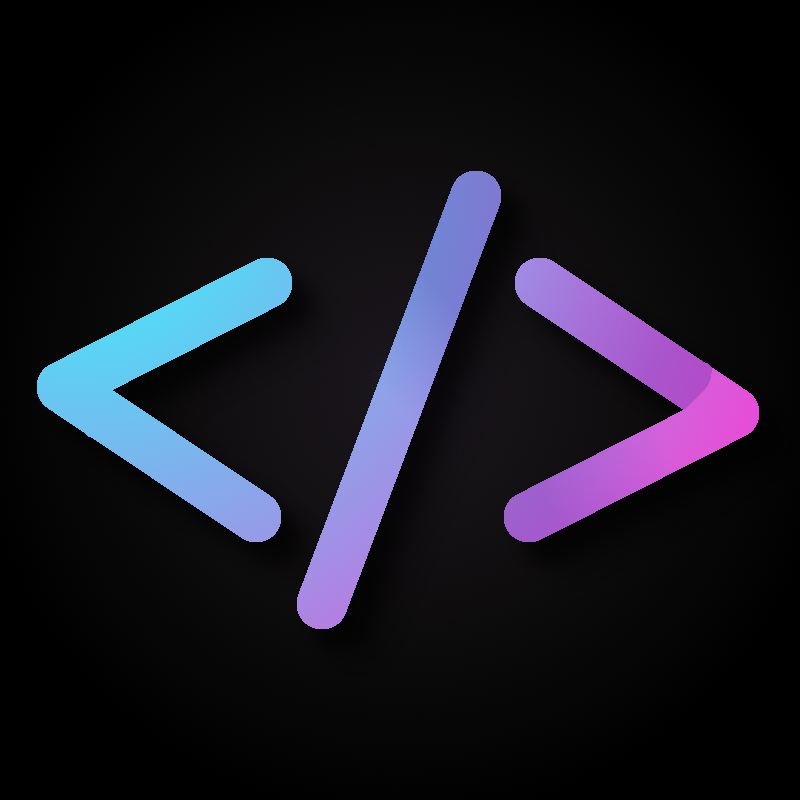
The article sure mentions 💩a lot.
The article sure mentions 💩a lot.
01.01.1970. Timestamp zero for the win.
No problem!
As an aside, I see we’re bringing the strangers thing over from Reddit. I hope more of the fun and funny stuff gets over, I miss some of the light shitposting.
Why not just cd $XDG_DOWNLOAD_DIR
in the first place?
did you mean smuts?
XDG specifies the capital names, but to be nitpickingly technically precise, linux systems don’t do this. It mostly is done by the distribution maintainers, and the XDG specs. A base system does not usually have a notion of anything beyond your $HOME.
Try adding a user: sudo adduser basicuser
. If you ls -al ~basicuser
you will see it’s almost empty, just the .bashrc (or in my fedora, there’s some .mozilla crap in /etc/skel that also gets bootstrapped).
ln -s Downloads downloads FTW
For bash, this is enough:
# Bash TAB-completition enhancements
# Case-insensitive
bind "set completion-ignore-case on"
# Treat - and _ as equivalent in tab-compl
bind "set completion-map-case on"
# Expand options on the _first_ TAB press.
bind "set show-all-if-ambiguous on"
If you also add e.g.CDPATH=~/Documents
, it will also always autocomplete from your Documents no matter which directory you’re on.
These technologies, although archaic, clumsy and insecure
Like cars? Or phones? Those are also archaic, clumsy and insecure technologies.
Sure -> I’m not smart enough to explain it like you’re five, but maybe 12 or so would work?
The problem here is that you’re not adding 1 + 2
, or 0.1 + 0.2
. You’re converting those to binary (because computers talk binary), then you’re adding binary numbers, and converting the result back. And the error happens at this conversion step. Let’s take it slow, one thing at a time.
See, if you are looking at decimal numbers, it’s kinda like this:
357 => 7 * 1 + 5 * 10 + 3 * 100. That sequence, from right to left, would be 1, 10, 100, … as you go from right to left, you keep multiplying that by 10.
Binary is similar, except it’s not 1, 10, 100, 1000 but rather 1, 2, 4, 8, 16 -> multiply by 2 instead of 10. So for example:
00101101 => right to left => 1 * 1 + 0 * 2 + 1 * 4 + 1 * 8 + 0 * 16 + 1 * 32 + 0 * 64 + 0 * 128 => 45
The numbers 0, 1, 2, 3…9 we call digits (since we can represent each of them with one digit). And the binary “numbers” 0 and 1 we call bits.
You can look up more at simple wikipedia links above probably.
We usually “align” these so that we fill with zeroes on the left until some sane width, which we don’t do in decimal.
132 is 132, right? But what if someone told you to write number 132 with 5 digits? We can just add zeroes. So call, “padding”.
00132 - > it’s the same as 132.
In computers, we often “align” things to 8 bits - or 8 places. Let’s say you have 5 - > 1001 in binary. To align it to 8 bits, we would add zeroes on the left, and write:
00001001 -> 1001 -> decimal 5.
Instead of, say, 100110, you would padd it to 8 bits, you can add two zeroes to left: 00100110.
Think of it as a thousands separator - we would not write down a million dollars like this: $1000000. We would more frequently write it down like this: $1,000,000, right? (Europe and America do things differently with thousands- and fractions- separators, so 1,000.00 vs 1.000,00. Don’t ask me why.)
So we group groups of three numbers usually, to have it easier to read large numbers.
E.g. 8487173209478 is hard to read, but 8 487 173 209 478 is simpler to see, it’s eight and a half trillion, right?
With binary, we group things into 8 bits - we call that “byte”. So we would often write this:
01000101010001001010101010001101
like this:
01000101 01000100 10101010 10001101
I will try to be using either 4 or 8 bits from now on, for binary.
As a tangential side note, we sometimes add “b” or “d” in front of numbers, that way we know if it’s decimal or binary. E.g. is 100 binary or decimal?
b100 vs d100 makes it easier. Although, we almost never use the d, but we do mark other systems that we use: b for binary, o for octal (system with 8 digits), h for hexadecimal (16 digits).
Anyway.
To convert numbers to binary, we’d take chunks out of it, write down the bit. Example:
13 -> ?
What we want to do is take chunks out of that 13 that we can write down in binary until nothing’s left.
We go from the biggest binary value and substract it, then go to next and next until we get that 13 down to zero. Binary values are 1, 2, 4, 8, 16, 32, … (and we write them down as b0001, b0010, b0100, b1000, … with more zeroes on the left.)
the biggest of those that fit into 13 seems to be 8, or 1000. So let’s start there. Our binary numbers so far: 1000 And we have 13 - 8 = 5 left to deal with.
The biggest binary to fit into 5 is 4 (b0100). Our binary so far: b1000 + b0100 And our decimal leftover: 5 - 4 = 1.
The biggest binary to fit into 1 is 1 (b0001). So binary: b1000 + b0100 + b0001 And decimal: 1 - 1 = 0.
So in the endl, we have to add these binary numbers:
b1101 `
So decimal 13 we write as 1101 in binary.
So far, so good, right? Let’s go to fractions now. It’s very similar, but we split parts before and after the dot.
E.g. 43.976 =>
Just note that we started already with 10 on the fractional part, not with 1 (so it’s 1/10, 1/100, 1/1000…)
The decimal part is similar, except instead of multiplying by 10, you divide by 10. It would be similar with binary: 1/2, 1/4, 1/8. Let’s try something:
b0101.0110 ->
So b0101.0110 (in binary) would be 5.375 in decimal.
Now, let’s convert 2.5 into binary, shall we?
First we take the whole part: 2. The biggest binary that fits is 2 (b0010). Now the fractional part, 0.5. What’s the biggest fraction we can write down? What are all of them?
If you remember, it’s 1/2, 1/4, 1/8, 1/16… or in other words, 0.5, 0.25, 0.125, 0.0625…
So 0.5 would be binary 1/2, or b0.1000
And finally, 2.5 in decimal => b0010.1000
Let’s try another one:
13.625
Together with b0.1000 above, it’s b0.1010 So the final number is:
b1101.1010
Get it? Try a few more:
4.125, 9.0625, 13.75.
Now, all these conversions so far, align very nicely. But what when they do not?
1 + 2 = 3. In binary, let’s padd it to 4 bits: 1 -> the biggest binary that fits is b0010. 2 -> the biggest thing that fits is b0010.
b0001 + b0010 = b0011.
If we convert the result back: b0011 -> to decimal, we get 3.
Okay? Good.
Now let’s try 0.1 + 0.2.
How do we get it in binary? Let’s find the biggest fraction that fits: 1/16, or 0.0625, or b0.0001
What’s left is 0.1 - 0.0625 = 0.0375.
Next binary that fits: 1/32 or 0.03125 or b0.00001. We’re left with 0.00625.
Next binary that fits is 1/256
… etc etc until we get to:
decimal 0.1 = b0.0001100110
We can do the same with 0.2 -> b0.0011001100.
Now, let’s add those two:
b0.0100 1100 10 `
Right? So far so good. Now, if we go back to decimal, it should come out to 0.3.
So let’s try it: 0/2+1/4+0/8+0/16+1/32+1/64+0/128+0/256+1/512+0/1024 => 0.298828125
WHAAAT?
There are several ways to achieve this. Also, “microfrontend” can mean many things. It’s usually referred to the architecture in which you can deploy an app shell, and other people or teams (or even companies) deploy their own, and you can include parts of their remote-deployed things into your live app. Look into Webpack 5 and Federated Modules for this. Look also into things like Single SPA. OR maybe Piral. There are other ways out there as well, but I haven’t tried them or they would not mix easily with Angular.
Then perhaps you mean deploy-time or build-time integration. From simple monorepos like nx.dev or workspace-like things, to even straight-forward publishing every module on npm. You can pack Angular Standalone Components into a package without issues. But even if you have older Angular versions like you mention, you can export web-component-like components and push them into a (private) npm namespace, and assemble on build time. And the same on react.
Then there are things like Astro, mentioned bellow, which kind of mix both of these ideas, and many more.
Your question is quite broad. If you have specific requirements, e.g. “I have a repo with two components, an angular app, two react apps in these versions, and I want to achieve X”, you may get specific recommendations, so fire away, ask questions.
I also didn’t think much of them, but when I compare this with off-the-shelf Synology or QNAP (in the consumer-grade, like I’m building), the Celeron is a beast :)
Are you sure? I mean the axe is a nice touch but did you edit the buffer before you smashed the PC?
Well can you attach it when you fill the 250 characters?
pata
How old are those? :)
Oh, I don’t want this to be a PC. I have plenty of CPU power for what I do, this has a different purpose.
I’m not planning to run anything much on those Celerons - it’s mostly just a file server. People do that with a RasPi - a 4-core CPU is going to blow it out of the water I think.
I also wanted some Ryzens, but my requirements were different. I did not want so much computational power, as much as I wanted low power. Combined with the price and availability, this works good enough for mne. We’ll see in the long run.
Yes, in fact! Two main reasons.
I wanted low-power, this is mostly gonna sit in the closet and serve files around. Even ARM CPUs like the RasPi can do that. But I didn’t want it to be too weak, in case I wanted a simple service or two, this still has extra oomph. This isn’t too powerful, but it is a 64-bit x86 CPU.
I also wanted some ports. This has 4 SATA ports. It’s supposed to be a NAS. It has a Gbit ethernet - I don’t have a Gbit network at home so this is good enough for now, and I can expand it somewhat. It has USBs, expansion slots etc.
those two combined resulted in a few selections, AsRock’s mini-ITX boards with integrated CPUs are quite good choices in this space.
I wanted low power consumption. I could have gone with a slightly stronger J5040-ITX perhaps, but it’s also using just slightly more power.
it’s also cheaper, the mobo with the CPU cost me 120€. The j5040 I mentioned would be a bit more - not a lot but still noticable.
I wanted silent, and this board and CPU is passively cooled. If I had money, I would get SSDs for storage as well (less power, less noise) but it’s a LOT more expensive.
I know there are other CPUs in this space but in the end you have to pick one so I did.
How did you find it for cable management?
[zlatko@dilj ~/Projects/galactic-bloodshed]$ man grep | wc -w 4297 [zlatko@dilj ~/Projects/galactic-bloodshed]$ man man | wc -w 4697 [zlatko@dilj ~/Projects/galactic-bloodshed]$